Adjust is an industry-leading mobile attribution provider that allows you to bring all your business data together to get powerful insights from it.
RudderStack supports Adjust as a destination to which you can seamlessly send your event data.
Getting started
Before configuring Adjust as a destination in RudderStack, verify if the source platform is supported by Adjust by referring to the table below:
Connection Mode | Web | Mobile | Server |
---|---|---|---|
Device mode | - | Supported | - |
Cloud mode | Supported | Supported | Supported |
Once you have confirmed that the platform supports sending events to Adjust, follow these steps:
- From your RudderStack dashboard, add the source. Then, from the list of destinations, select Adjust.
- Assign a name to the destination and click Continue.
Connection settings
To successfully configure Adjust as a destination, you will need to configure the following settings:
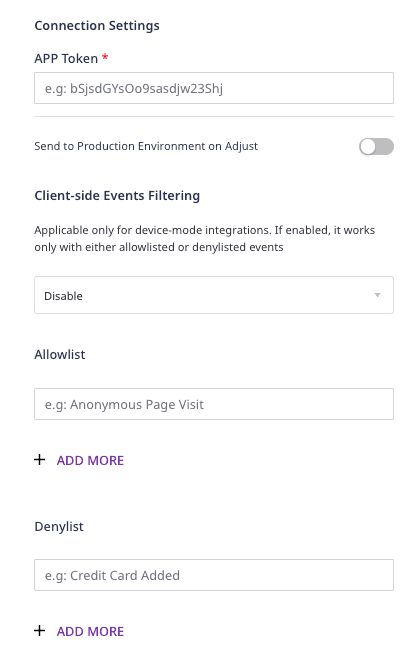
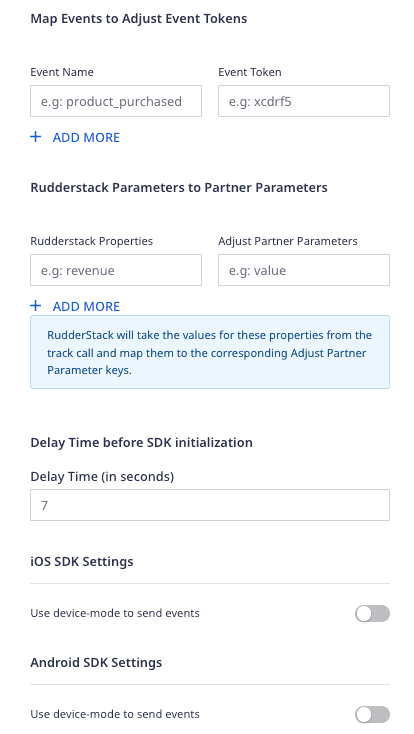
- APP Token: Enter your Adjust app token.
- Send to Production Environment on Adjust: Enable this setting to send the data to the production environment in Adjust. By default, RudderStack sends the data to the Adjust sandbox environment.
- Client-side Events Filtering: This setting lets you specify which events should be blocked or allowed to flow through to Adjust.
- Map events to Adjust Event Tokens: This setting lets you map the RudderStack events to Adjust's event tokens.
- RudderStack Parameters to Partner Parameters: This setting lets you map your event properties to specific Adjust partner parameters.
- Delay Time before SDK initialization: This setting is applicable only for sending events via the device mode. You can use it to initiate a delay in loading the SDK for the first time.
- Use device mode to send events: Enable this setting for the Android/iOS SDK to send events to Adjust via the device mode.
Adding device mode integration
Follow the below steps to add Adjust to your project depending on your integration platform:
- Add the following line to your CocoaPods
Podfile
:pod 'Rudder-Adjust' - After adding the dependency, register the
RudderAdjustFactory
with yourRudderClient
initialization as afactory
ofRudderConfig
. Run the following command to importRudderAdjustFactory.h
file in yourAppDelegate.m
file:#import <Rudder-Adjust/RudderAdjustFactory.h> - Then, change the SDK initialization to the following:RudderConfigBuilder *builder = [[RudderConfigBuilder alloc] init];[builder withDataPlaneUrl:DATA_PLANE_URL];[builder withFactory:[RudderAdjustFactory instance]];[RudderClient getInstance:WRITE_KEY config:[builder build]];
- Install
RudderAdjust
(available through CocoaPods) by adding the following line to yourPodfile
:pod 'RudderAdjust', '~> 1.0.0' - Run the
pod install
command. - Next, import the SDK depending on your preferred platform:import RudderAdjust@import RudderAdjust;
- Add the imports to your
AppDelegate
file under thedidFinishLaunchingWithOptions
method, as shown:let config: RSConfig = RSConfig(writeKey: WRITE_KEY).dataPlaneURL(DATA_PLANE_URL)RSClient.sharedInstance().configure(with: config)RSClient.sharedInstance().addDestination(RudderAdjustDestination())RSConfig *config = [[RSConfig alloc] initWithWriteKey:WRITE_KEY];[config dataPlaneURL:DATA_PLANE_URL];[[RSClient sharedInstance] configureWith:config];[[RSClient sharedInstance] addDestination:[[RudderAdjustDestination alloc] init]];
- Add
mavenCentral()
to therepositories
section of yourbuild.gradle
file:repositories {mavenCentral()} - Next, add the following permissions to your
AndroidManifest.xml
file:<uses-permission android:name="android.permission.INTERNET"/><uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>// If you are not targeting the Google Play Store, you need to add the following permission:<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/>// If you are targeting Android 13 and above (API level 33), you need to add the com.google.android.gms.AD_ID permission to read the device's advertising ID.<uses-permission android:name="com.google.android.gms.permission.AD_ID"/> - Finally, add the following lines in your
build.gradle
file underdependencies
:// RudderStack Android-SDKimplementation 'com.rudderstack.android.sdk:core:[1.0,2.0)'// RudderStack Adjust-SDKimplementation 'com.rudderstack.android.integration:adjust:1.0.1'// Add Google Play Services library to enable the Google Advertising ID for Adjust SDKimplementation 'com.google.android.gms:play-services-ads-identifier:17.0.1'// To support the Google Play Referrer API, make sure you have the following in your build.gradle file:implementation 'com.android.installreferrer:installreferrer:2.2'For more information on the implementationcom.google.android.gms:play-services-ads-identifier:17.0.1
, refer to the Adjust documentation. - After adding the dependency, register the
RudderAdjustFactory
with yourRudderClient
initialization as afactory
ofRudderConfig
. Add the following line in yourApplication
class:import com.rudderstack.android.integration.adjust.AdjustIntegrationFactory; - Finally, change the SDK initialization to the following:val rudderClient: RudderClient = RudderClient.getInstance(this,WRITE_KEY,RudderConfig.Builder().withDataPlaneUrl(DATA_PLANE_URL).withFactory(AdjustIntegrationFactory.FACTORY).build())
- First, add the RudderStack Unity SDK to your project.
- Download the Adjust SDK extension package and import it in your project.The package comes with Adjust Unity SDK embedded in it along with the required
jar
files for Android Install Referrer. It is strongly recommended to not add the Adjust SDK separately. - After importing the
rudder-unity-extension-adjust.unitypackage
to your project, attach theRudderPreferbs.prefab
file fromRudderUnityPlugin
to your mainGameObject
. - Finally, change the SDK initialization, as shown:// Build your configRudderConfigBuilder configBuilder = new RudderConfigBuilder().WithEndPointUrl(DATA_PLANE_URL).WithFactory(RudderAdjustIntegrationFactory.GetFactory());// Get instance for RudderClientRudderClient rudderClient = RudderClient.GetInstance(WRITE_KEY,configBuilder.Build());
- Add the following dependency to the
dependencies
section of yourpubspec.yaml
file.rudder_integration_adjust_flutter: ^1.0.1 - Run the below command to install the dependency added in the above step:flutter pub get
- Import the
RudderIntegrationAdjustFlutter
in your application where you are initializing the SDK.import 'package:rudder_integration_adjust_flutter/rudder_integration_adjust_flutter.dart'; - Finally, change the initialization of your
RudderClient
as shown:final RudderController rudderClient = RudderController.instance;RudderConfigBuilder builder = RudderConfigBuilder();builder.withFactory(RudderIntegrationAdjustFlutter());rudderClient.initialize(<WRITE_KEY>, config: builder.build(), options: null);
Identify
RudderStack's identify
call lets you identify a visiting user and associate them to their actions.
RudderStack sends the user information in the identify
call to Adjust's addSessionPartnerParameter
method to set the userId
(or anonymousId
, in case userId
is absent), so that the user information is passed to the subsequent calls.
A sample identify
call is shown below:
[[RudderClient sharedInstance] identify:@"developer_user_id" traits:@{@"foo": @"bar", @"foo1": @"bar1"}];
Track
The track
call lets you record the user actions along with any properties associated with them.
When you make a track
call, RudderStack maps the event name with the corresponding Adjust custom event in the dashboard using Adjust's trackEvent
method.
Sending callback parameters to Adjust
RudderStack sends all the custom properties in your track
calls as callback parameters.
A sample track
call is shown below:
[[RudderClient sharedInstance] track:@"test_event" properties:@{@"key":@"value", @"foo": @"bar"}];
Sending partner parameters to Adjust
You can also send custom properties in your track
calls as partner parameters to Adjust. Adjust then sends those parameters to the external partners you have set up in your Adjust dashboard.
RudderStack uses the property mappings specified in the RudderStack Parameters to Partner Parameters setting to check if a key is present in the track
event properties and maps it to the corresponding Adjust partner parameter object.
Suppose a customer sets the following mapping in the RudderStack dashboard:
RudderStack property | Adjust partner parameter |
---|---|
revenue | price |
quantity | quantity |
A sample track
call with the above properties is shown below:
[[RudderClient sharedInstance] track:@"purchase" properties:@{@"revenue":@20.99, @"currency": @"USD", @"quantity": @10}];
The corresponding Adjust payload highlighting the parameters is shown below:
"params": { "android_id": "3f034872-5e28-45a1-9eda-ce22a3e36d1a", "gps_adid": "3f034872-5e28-45a1-9eda-ce22a3e36d1a", "att_status": 3, "tracking_enabled": true, "currency": "USD", "ip_address": "[::1]", "s2s": 1, "app_token": "t1yurrb968zk", "event_token": "tf4gm5", "environment": "production", "partner_params": {"price":"20.99","quantity":"10"}},
Sending revenue tracking events to Adjust
To send revenue tracking events to Adjust, your can add total
and currency
to your event properties, as shown:
[[RudderClient sharedInstance] track:@"purchase" properties:@{@"total":@2.99, @"currency": @"USD"}];
Reset
You can use RudderStack's reset
method to call resetSessionCallbackParameters
of the Adjust SDK to reset the user ID and the associated traits.
A sample reset
call is shown below:
[[RudderClient sharedInstance] reset];
App install attribution
Adjust's native SDK handles app install attribution out of the box with RudderStack.
Adjust environment dependency on log level
RudderStack sends data to the Adjust environment depending on the RudderLogLevel
set in the SDK, as listed in the below table:
RudderLogLevel | Adjust Environment | Adjust SDK LogLevel |
---|---|---|
DEBUG / VERBOSE | Sandbox | VERBOSE |
NONE / ERROR / WARN / INFO | Production | ERROR |
FAQ
Where can I find the Adjust app token?
To get your Adjust app token, follow these steps:
- Log into your Adjust dashboard.
- Find your app and select the app options caret (^), as shown:
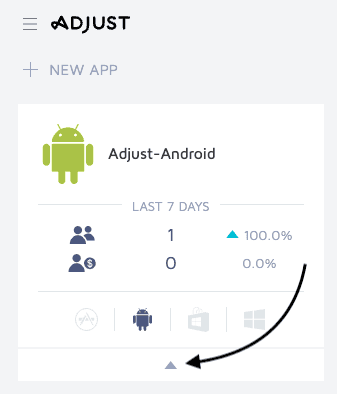
- You will find your app token listed here.
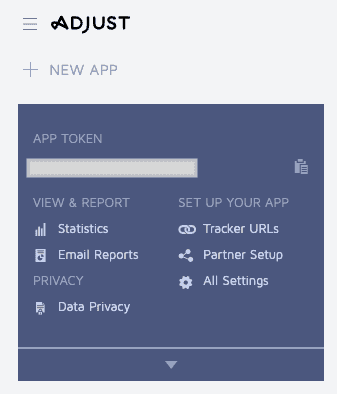
How can I create a new event token in Adjust?
To create a new event token, follow these steps:
- Log into your Adjust dashboard.
- Find your app and select the app options caret (^), as shown:
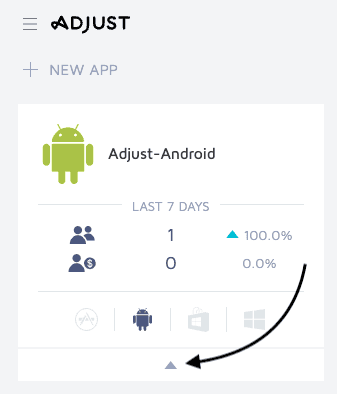
- Go to All Settings > Events, as shown:
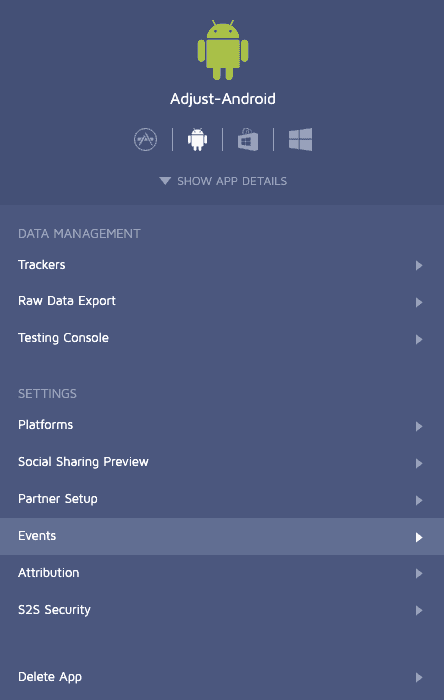
- Under CREATE NEW EVENT, enter the name of the event token and click CREATE.
How can I set up new partners in Adjust?
Adjust lets you provide additional data to certain integrated partners. To set up a new partner in Adjust, follow these steps:
- Log into your Adjust dashboard.
- Find your app and select the app options caret (^), as shown:
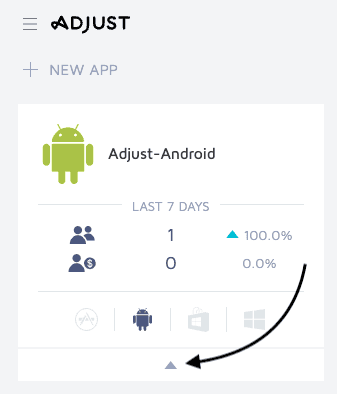
- Go to All Settings > Partner Setup > ADD PARTNERS, as shown:
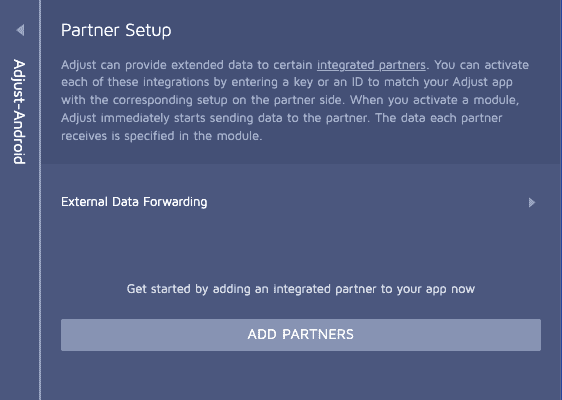
- Select the partner from the list and click the + option on the right to add.
- Enter the relevant details to complete the configuration and click SAVE to complete the setup.
Contact us
For more information on the topics covered on this page, email us or start a conversation in our Slack community.